Input
POST https://gateway.appypie.com/sdxl/getImageTurbo/v1/SDXL_Turbo_TextImage HTTP/1.1 Content-Type: application/json Cache-Control: no-cache { "prompt": "Generate a detailed futuristic cityscape at sunset with towering skyscrapers, flying vehicles, and vibrant orange and purple skies. Blend modern architecture with natural elements like parks and flowing water, capturing a tranquil yet innovative atmosphere.", "negative_prompt": "Exclude low-quality, blurry, or pixelated details. Avoid dull colors, overly simplistic architecture, empty streets, or outdated technology. Omit any chaotic or disorganized elements, dark or gloomy tones, and unnatural lighting.", "height": 768, "width": 768, "num_inference_steps": 20, "guidance_scale": 5, "seed": 20 }
import urllib.request, json try: url = "https://gateway.appypie.com/sdxl/getImageTurbo/v1/SDXL_Turbo_TextImage" hdr ={ # Request headers 'Content-Type': 'application/json', 'Cache-Control': 'no-cache', } # Request body data = data = json.dumps(data) req = urllib.request.Request(url, headers=hdr, data = bytes(data.encode("utf-8"))) req.get_method = lambda: 'POST' response = urllib.request.urlopen(req) print(response.getcode()) print(response.read()) except Exception as e: print(e)
// Request body const body = { "prompt": "Generate a detailed futuristic cityscape at sunset with towering skyscrapers, flying vehicles, and vibrant orange and purple skies. Blend modern architecture with natural elements like parks and flowing water, capturing a tranquil yet innovative atmosphere.", "negative_prompt": "Exclude low-quality, blurry, or pixelated details. Avoid dull colors, overly simplistic architecture, empty streets, or outdated technology. Omit any chaotic or disorganized elements, dark or gloomy tones, and unnatural lighting.", "height": 768, "width": 768, "num_inference_steps": 20, "guidance_scale": 5, "seed": 20 } ; fetch('https://gateway.appypie.com/sdxl/getImageTurbo/v1/SDXL_Turbo_TextImage', { method: 'POST', body: JSON.stringify(body), // Request headers headers: { 'Content-Type': 'application/json', 'Cache-Control': 'no-cache',} }) .then(response => { console.log(response.status); console.log(response.text()); }) .catch(err => console.error(err));
curl -v -X POST "https://gateway.appypie.com/sdxl/getImageTurbo/v1/SDXL_Turbo_TextImage" -H "Content-Type: application/json" -H "Cache-Control: no-cache" --data-raw "{ \"prompt\": \"Generate a detailed futuristic cityscape at sunset with towering skyscrapers, flying vehicles, and vibrant orange and purple skies. Blend modern architecture with natural elements like parks and flowing water, capturing a tranquil yet innovative atmosphere.\", \"negative_prompt\": \"Exclude low-quality, blurry, or pixelated details. Avoid dull colors, overly simplistic architecture, empty streets, or outdated technology. Omit any chaotic or disorganized elements, dark or gloomy tones, and unnatural lighting.\", \"height\": 768, \"width\": 768, \"num_inference_steps\": 20, \"guidance_scale\": 5, \"seed\": 20 }"
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import java.util.HashMap; import java.util.Map; import java.io.UnsupportedEncodingException; import java.io.DataInputStream; import java.io.InputStream; import java.io.FileInputStream; public class HelloWorld { public static void main(String[] args) { try { String urlString = "https://gateway.appypie.com/sdxl/getImageTurbo/v1/SDXL_Turbo_TextImage"; URL url = new URL(urlString); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); //Request headers connection.setRequestProperty("Content-Type", "application/json"); connection.setRequestProperty("Cache-Control", "no-cache"); connection.setRequestMethod("POST"); // Request body connection.setDoOutput(true); connection .getOutputStream() .write( "{ \"prompt\": \"Generate a detailed futuristic cityscape at sunset with towering skyscrapers, flying vehicles, and vibrant orange and purple skies. Blend modern architecture with natural elements like parks and flowing water, capturing a tranquil yet innovative atmosphere.\", \"negative_prompt\": \"Exclude low-quality, blurry, or pixelated details. Avoid dull colors, overly simplistic architecture, empty streets, or outdated technology. Omit any chaotic or disorganized elements, dark or gloomy tones, and unnatural lighting.\", \"height\": 768, \"width\": 768, \"num_inference_steps\": 20, \"guidance_scale\": 5, \"seed\": 20 }".getBytes() ); int status = connection.getResponseCode(); System.out.println(status); BufferedReader in = new BufferedReader( new InputStreamReader(connection.getInputStream()) ); String inputLine; StringBuffer content = new StringBuffer(); while ((inputLine = in.readLine()) != null) { content.append(inputLine); } in.close(); System.out.println(content); connection.disconnect(); } catch (Exception ex) { System.out.print("exception:" + ex.getMessage()); } } }
$url = "https://gateway.appypie.com/sdxl/getImageTurbo/v1/SDXL_Turbo_TextImage"; $curl = curl_init($url); curl_setopt($curl, CURLOPT_CUSTOMREQUEST, "POST"); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); # Request headers $headers = array( 'Content-Type: application/json', 'Cache-Control: no-cache',); curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); # Request body $request_body = '{ "prompt": "Generate a detailed futuristic cityscape at sunset with towering skyscrapers, flying vehicles, and vibrant orange and purple skies. Blend modern architecture with natural elements like parks and flowing water, capturing a tranquil yet innovative atmosphere.", "negative_prompt": "Exclude low-quality, blurry, or pixelated details. Avoid dull colors, overly simplistic architecture, empty streets, or outdated technology. Omit any chaotic or disorganized elements, dark or gloomy tones, and unnatural lighting.", "height": 768, "width": 768, "num_inference_steps": 20, "guidance_scale": 5, "seed": 20 } '; curl_setopt($curl, CURLOPT_POSTFIELDS, $request_body); $resp = curl_exec($curl); curl_close($curl); var_dump($resp);
Output
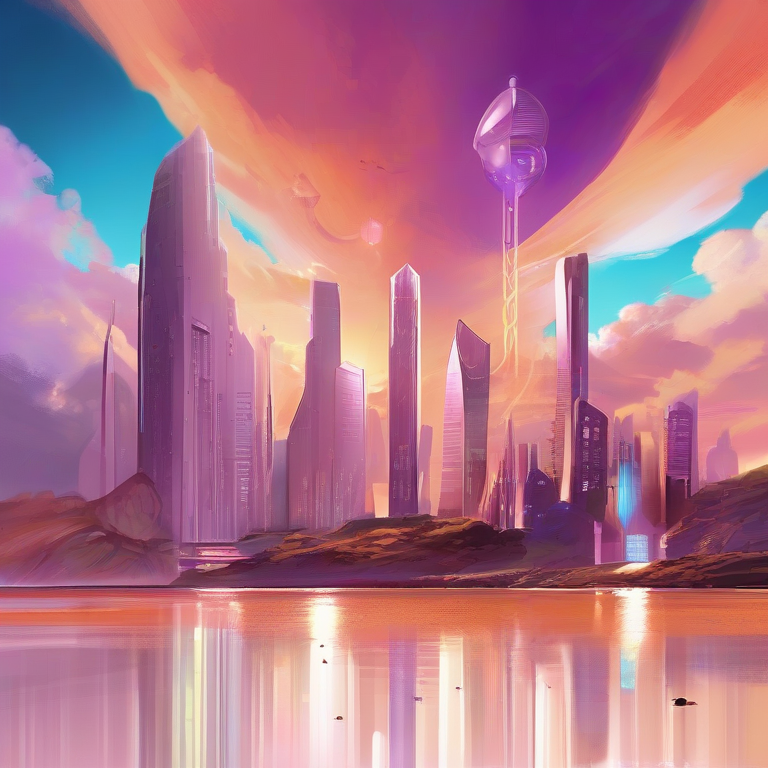
Top Trending Generative AI APIs
Maximize the potential of your projects with our Generative AI APIs. From video generation & image creation to text generation, animation, 3D modeling, prompt generation, image restoration, and code generation, our advanced APIs cover all aspects of generative AI to meet your needs.