Input
POST https://gateway.appypie.com/getImage/v1/getSDXLImage HTTP/1.1 Content-Type: application/json Cache-Control: no-cache { "prompt": "High-resolution, realistic image of a sparrow bird perched on a blooming cherry blossom branch during springtime. The sparrow's feathers should be finely detailed with natural colors, including shades of brown and white. The background should be soft-focused with a clear blue sky, creating a serene and peaceful atmosphere.", "negative_prompt": "Low-quality, blurry image, with any other birds or animals. Avoid abstract or cartoonish styles, dark or gloomy atmosphere, unnecessary objects or distractions in the background, harsh lighting, and unnatural colors.", "height": 1024, "width": 1024, "num_steps": 20, "guidance_scale": 5, "seed": 40 }
import urllib.request, json try: url = "https://gateway.appypie.com/getImage/v1/getSDXLImage" hdr ={ # Request headers 'Content-Type': 'application/json', 'Cache-Control': 'no-cache', } # Request body data = data = json.dumps(data) req = urllib.request.Request(url, headers=hdr, data = bytes(data.encode("utf-8"))) req.get_method = lambda: 'POST' response = urllib.request.urlopen(req) print(response.getcode()) print(response.read()) except Exception as e: print(e)
// Request body const body = { "prompt": "High-resolution, realistic image of a sparrow bird perched on a blooming cherry blossom branch during springtime. The sparrow's feathers should be finely detailed with natural colors, including shades of brown and white. The background should be soft-focused with a clear blue sky, creating a serene and peaceful atmosphere.", "negative_prompt": "Low-quality, blurry image, with any other birds or animals. Avoid abstract or cartoonish styles, dark or gloomy atmosphere, unnecessary objects or distractions in the background, harsh lighting, and unnatural colors.", "height": 1024, "width": 1024, "num_steps": 20, "guidance_scale": 5, "seed": 40 }; fetch('https://gateway.appypie.com/getImage/v1/getSDXLImage', { method: 'POST', body: JSON.stringify(body), // Request headers headers: { 'Content-Type': 'application/json', 'Cache-Control': 'no-cache',} }) .then(response => { console.log(response.status); console.log(response.text()); }) .catch(err => console.error(err));
curl -v -X POST "https://gateway.appypie.com/getImage/v1/getSDXLImage" -H "Content-Type: application/json" -H "Cache-Control: no-cache" --data-raw "{ \"prompt\": \"High-resolution, realistic image of a sparrow bird perched on a blooming cherry blossom branch during springtime. The sparrow's feathers should be finely detailed with natural colors, including shades of brown and white. The background should be soft-focused with a clear blue sky, creating a serene and peaceful atmosphere.\", \"negative_prompt\": \"Low-quality, blurry image, with any other birds or animals. Avoid abstract or cartoonish styles, dark or gloomy atmosphere, unnecessary objects or distractions in the background, harsh lighting, and unnatural colors.\", \"height\": 1024, \"width\": 1024, \"num_steps\": 20, \"guidance_scale\": 5, \"seed\": 40 }"
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import java.util.HashMap; import java.util.Map; import java.io.UnsupportedEncodingException; import java.io.DataInputStream; import java.io.InputStream; import java.io.FileInputStream; public class HelloWorld { public static void main(String[] args) { try { String urlString = "https://gateway.appypie.com/getImage/v1/getSDXLImage"; URL url = new URL(urlString); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); //Request headers connection.setRequestProperty("Content-Type", "application/json"); connection.setRequestProperty("Cache-Control", "no-cache"); connection.setRequestMethod("POST"); // Request body connection.setDoOutput(true); connection .getOutputStream() .write( "{ \"prompt\": \"High-resolution, realistic image of a sparrow bird perched on a blooming cherry blossom branch during springtime. The sparrow's feathers should be finely detailed with natural colors, including shades of brown and white. The background should be soft-focused with a clear blue sky, creating a serene and peaceful atmosphere.\", \"negative_prompt\": \"Low-quality, blurry image, with any other birds or animals. Avoid abstract or cartoonish styles, dark or gloomy atmosphere, unnecessary objects or distractions in the background, harsh lighting, and unnatural colors.\", \"height\": 1024, \"width\": 1024, \"num_steps\": 20, \"guidance_scale\": 5, \"seed\": 40 }".getBytes() ); int status = connection.getResponseCode(); System.out.println(status); BufferedReader in = new BufferedReader( new InputStreamReader(connection.getInputStream()) ); String inputLine; StringBuffer content = new StringBuffer(); while ((inputLine = in.readLine()) != null) { content.append(inputLine); } in.close(); System.out.println(content); connection.disconnect(); } catch (Exception ex) { System.out.print("exception:" + ex.getMessage()); } } }
$url = "https://gateway.appypie.com/getImage/v1/getSDXLImage"; $curl = curl_init($url); curl_setopt($curl, CURLOPT_CUSTOMREQUEST, "POST"); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); # Request headers $headers = array( 'Content-Type: application/json', 'Cache-Control: no-cache',); curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); # Request body $request_body = '{ "prompt": "High-resolution, realistic image of a sparrow bird perched on a blooming cherry blossom branch during springtime. The sparrow's feathers should be finely detailed with natural colors, including shades of brown and white. The background should be soft-focused with a clear blue sky, creating a serene and peaceful atmosphere.", "negative_prompt": "Low-quality, blurry image, with any other birds or animals. Avoid abstract or cartoonish styles, dark or gloomy atmosphere, unnecessary objects or distractions in the background, harsh lighting, and unnatural colors.", "height": 1024, "width": 1024, "num_steps": 20, "guidance_scale": 5, "seed": 40 }'; curl_setopt($curl, CURLOPT_POSTFIELDS, $request_body); $resp = curl_exec($curl); curl_close($curl); var_dump($resp);
Output
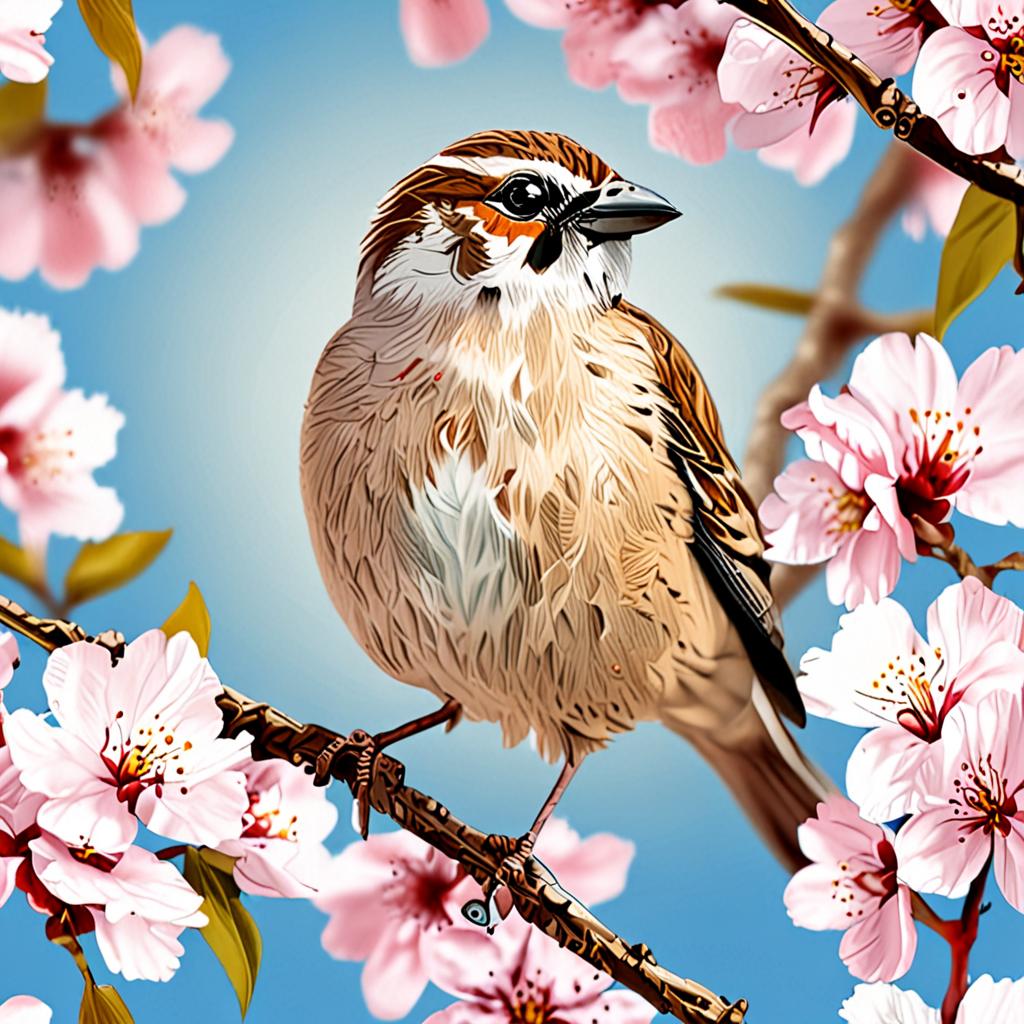
Pricing
This image generation API is offered on a monthly subscription basis.
Basic$19/month
- 5,000 API calls/month
- Personalized API key
- API Documentation
- Personalized support
- Cancel anytime
Standard$49/month
- 15,000 API calls/month
- $0.004 per API calls after exceeding the monthly limit.
- Personalized API key
- API Documentation
- Personalized support
- Cancel anytime
Pro$99/month
- 40,000 API calls/month
- $0.0035 per API calls after exceeding the monthly limit.
- Personalized API key
- API Documentation
- Personalized support
- Cancel anytime
Top Trending Generative AI APIs
Maximize the potential of your projects with our Generative AI APIs. From video generation & image creation to text generation, animation, 3D modeling, prompt generation, image restoration, and code generation, our advanced APIs cover all aspects of generative AI to meet your needs.